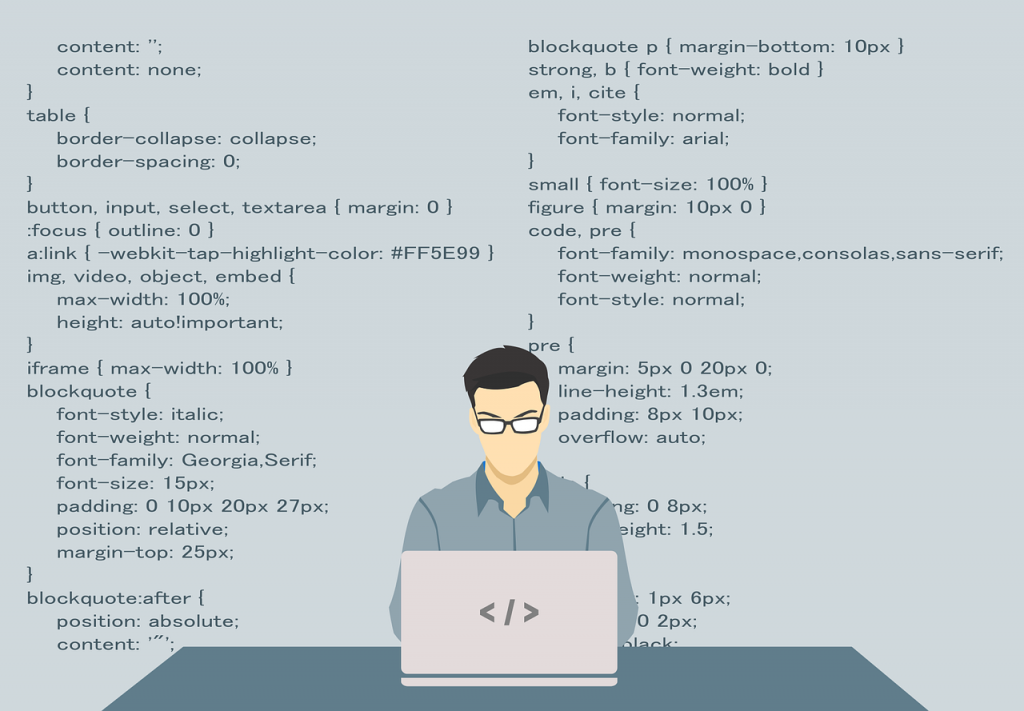
It doesn’t matter how well your application is designed, errors always remain in some way or the other. Errors may be of many types: Runtime errors, Operational errors or System errors. It is very important that we should write Error handling Code in the application so that the application handles the errors smoothly and recovers the lost data due to errors. VB and JAVA both provide a convenient way of handling errors. Let us first examine the error handling code structure in JAVA.
Exception Handling in JAVA
Errors in JAVA are called ‘Exceptions’. There are various classes in JAVA which provide an interface to handle exceptions. But the important point to keep in mind is that the code for which you want to handle the exception should be enclosed within ‘try’ and ‘catch’ statements. Below is the structure of writing a exception handling code.
try {
(code here)
}catch (ExceptionType e) {
(exception handling code)
}
From the above example, you can clearly get an idea of how exception handling works in JAVA. In place of ‘exceptionType’, you will declare a variable of type of the exception that you want to handle.
Example
Let us illustrate it with an example. Suppose, you want to divide a number. So you declare two variables ‘a’ and ‘b’ and divide ‘a’ by ‘b’. Now further suppose that you assign values to ‘a’ and ‘b’. For ‘a’, you provide 20 and for ‘b’, you provide 0. Now, you must be wondering that I am a fool i.e. why I am trying to divide 20 by 0. But I am doing this because I want the program to invoke an exception while dividing 20 by 0. In JAVA, there is an exception class which handles Arithmetic operational exceptions. This class is called ‘ArithmeticException’. So, with the help of this class, we will write a program with exception handling as follows:
public void myFunction() {
try {
int a = 20;
int b = 0;
int c = a / b;
System.out.print("Answer = " + c);
}
catch (ArithmeticException e) {
System.out.print(e.getMessage());
}
}
In the above example, the program first tries to divide ‘a’ by ‘b’ in the try block. When it gets an arithmetic exception while calculation, it quickly jumps to the catch block and displays an exception message as ‘/ by 0‘ in the console window. It uses getMessage()
method of the exception type object to display an error message.
So this is how exception handling works in JAVA. Now, we will have a look on how exception handling works in VB.
Error Handling in VB
In VB, errors are simply called ‘Errors’. But error handling in VB is a little different than JAVA. In VB, error handling statement is placed at the start of the function and error handling code is placed at the end of the function. Below is the structure of writing an error handling program in VB.
Function myFunction()
On Error GoTo ErrHandler
(code here)
Exit Function
ErrHandler:
(error handling code)
End Function
From the above example, you can notice that error handling approach in VB is a little bit different from JAVA. In the first statement, you have to provide an address link to the section where error handling code is written. In the above example, ‘ErrHandler
‘ is the name of the error handling section which is placed at the bottom of the function after ‘Exit Sub
‘ statement. ‘Exit Sub
‘ plays a role of ending the function if the function exits without facing any errors. When the function starts, it performs the operation and then exits the function. But if it gets an error during operation, it leaves everything and jumps to ‘ErrHandler
‘ section at the end and performs the error handling code written there.
Example
Now, we shall experiment the above calculation example written in JAVA which threw an Arithmetic Exception with VB. The above example can be wriitten in VB as follows:
Function myFunction()
On Error GoTo calcErrHandler
Dim a as Integer, b as Integer, c as Integer
a = 20
b = 0
c = a / b
Msgbox "Answer = " & c
Exit Function
calcErrHandler:
Msgbox Err.Description
End Function
In the above example, you can see that the function first initializes variables ‘a
‘, ‘b
‘ and ‘c
‘. Then assigns value 20 to ‘a
‘ and 0 to ‘b
‘. Then, calculates ‘c
‘ as a / b
. Now, here the program invokes an error and jumps to ‘calcErrHandler
‘ and displays a message box which says ‘Division by 0’. VB has an ‘Err
‘ object which provides functions to handle errors. We are using the ‘Description
‘ property of this ‘Err
‘ object to display the error message.
Another way of handling errors in VB
There is also an another way of handling errors in VB. If you want that the program should not get interrupted even though if an error occurs, you can use a statement ‘On Error Resume Next
‘ instead of ‘On Error GoTo calcErrHandler
‘. which offers an uninterrupted process even though if an error occurs. In this case, if an error occurs, the program executes the next line without interrupting the program. For e.g. the above example can be re-written again as follows:
Function myFunction()
On Error Resume Next
Dim a as Integer, b as Integer, c as Integer
a = 20
b = 0
c = a / b
Msgbox "Answer = " & c
End Function
The function above doesn’t invoke an error. Instead, it divides ‘a
‘ by ‘b
‘ and calculates a value equal to 0. Then it shows a message box displaying a message ‘Answer=0’.
This way your program will never get interrupted due to errors but VB will make the automatic adjustments to the variables which are affected due to errors. in the above example, VB compiler faced a problem in dividing 20 by 0. So instead of showing an error, it automatically adjusted the result and assigned 0 to ‘c
‘.