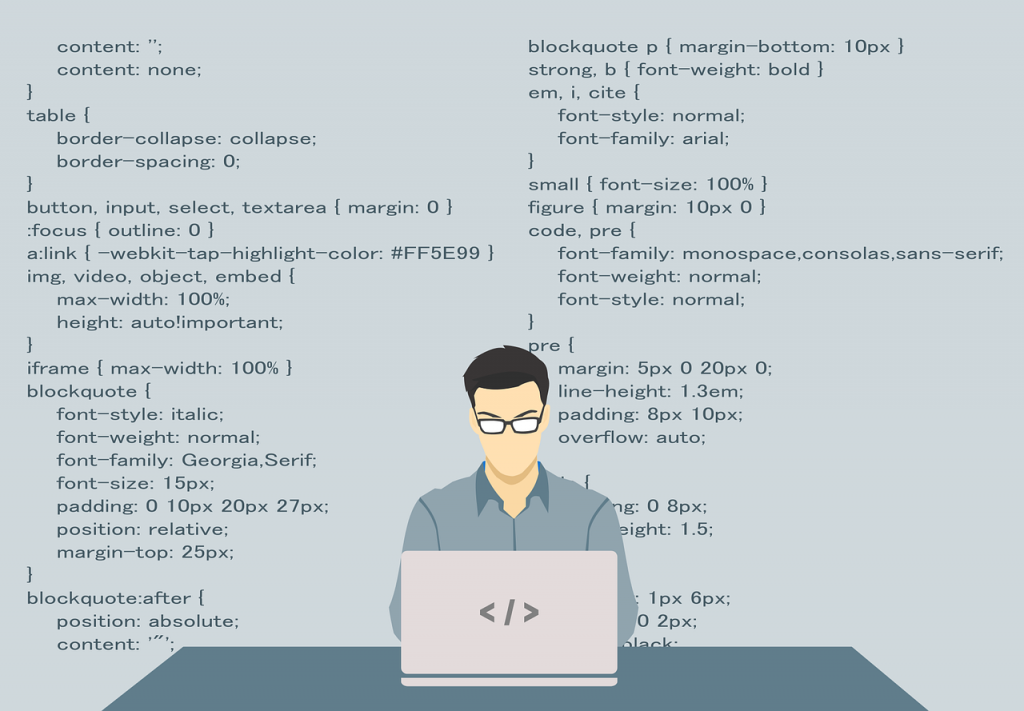
VB and Java have a significant difference in their basic syntax rules. But the major difference between the two is that JAVA is a case-sensitive language whereas VB is not a case-sensitive language. That means that the words ‘ if ‘ and ‘ If ‘ are the same in VB but they are not the same in JAVA and hence, JAVA compiler will show an error if you write ‘ If ‘ instead of ‘ if ‘. The second major difference is that in Java, every command line is ended with a semi-colon (;) whereas in VB, no symbol is used to end a line. Simply press ‘Enter’ in VB and the line is complete.
Variable Declaration
In Java, it is important that you declare a variable first before it can be used. Whereas in VB, if you don’t declare a variable before using it, it will not show any compiler errors but it will treat the variable as variant. But if you want that like Java, it should show errors if an undeclared variable is used, just place ‘Option Explicit’ command at the beginning of your module. Once you do this, VB compiler will show an error if an undeclared variable is used. Below is the list of the declaration syntax of some of the common variable types used in both VB and JAVA.
VBDim i as Integer
Javaint i
VBDim s as String
JavaString s
VBDim d as Double
Javadouble d
VBDim f as Single
Javafloat f
VBDim l as Long
Javalong l
Following is a detailed description of some of the major differences between the syntax of some of the major commands used in VB and Java.
For Loop
You can notice in the above example that there is a considerable difference between VB and JAVA For Loops. The above example loops and performs user defined operation on data 12 times. In Java, there is a provision if you don’t want to declare a variable before using it in for loop. Replace the line ‘for (i = 0; i <12; i++)
‘ with ‘for (int i = 0; i < 12; i++)
‘. This statement declares ‘int i
‘first and then uses it in for loop. Therefore, with this approach, you don’t need to write a declaration line ‘int i;
‘.
Do Loop
The Do Loop is quite similar in both the languages. But in VB, there are two ways of writing a do loop. One is written above and the other one is as follows:
Do While (condition)<
(processing here)
Loop
The difference between the two is that the first one checks the looping at the end of the loop whereas the second loop checks the looping at the beginning of the loop.
IF statement
You can notice than in VB, ‘If
‘ statement is followed by a keyword ‘Then
‘ whereas in Java, there is no such keyword. The body of the Java ‘if
‘ and ‘else
‘ statements is enclosed by braces (‘{}’) whereas the body of the VB ‘if
‘ and ‘else
‘ statement is enclosed between the keywords ‘Then
‘ and ‘End If
‘.
Select statement
In Java, ‘Select
‘ statement is called ‘Switch
‘ statement.
Functions in VB, Methods in JAVA
Every programming language provides a capability to the developer of writing routines to perform various operations. Such routines in JAVA are called ‘Methods’ and in VB, they are called ‘Functions’. The proper way of writing them in both the languages is as follows:
The routines above are simple and don’t return a value. But consider a situation where you want that some operation is performed in a routine and then, a value is returned. For e.g.: If you want to write a routine which takes to numbers as parameters and calculates and returns their sum, you can do it as follows:
From the above example, you can notice that though the calculation code is almost the same but the way of returning a value from the function is different. In VB, function’s name is assigned the value that is to return whereas in JAVA, a return statement is used to return a value. In order to make a function value returnable in VB, use ‘as
(Variable type)’ keywords post-fixed to the function’s name. For e.g. for writing a function which returns an Integer, use ‘Function myFunc() as Integer
‘. If you don’t want to return any value, simply don’t post-fix anything. In Java, ‘void
(meaning no value)’ keyword is used in a method if you don’t want to return any value. For returning a value, simply specify the returning variable type in place of ‘ void’. For e.g. ‘public int myFunction()
‘ in order to return an Integer value.